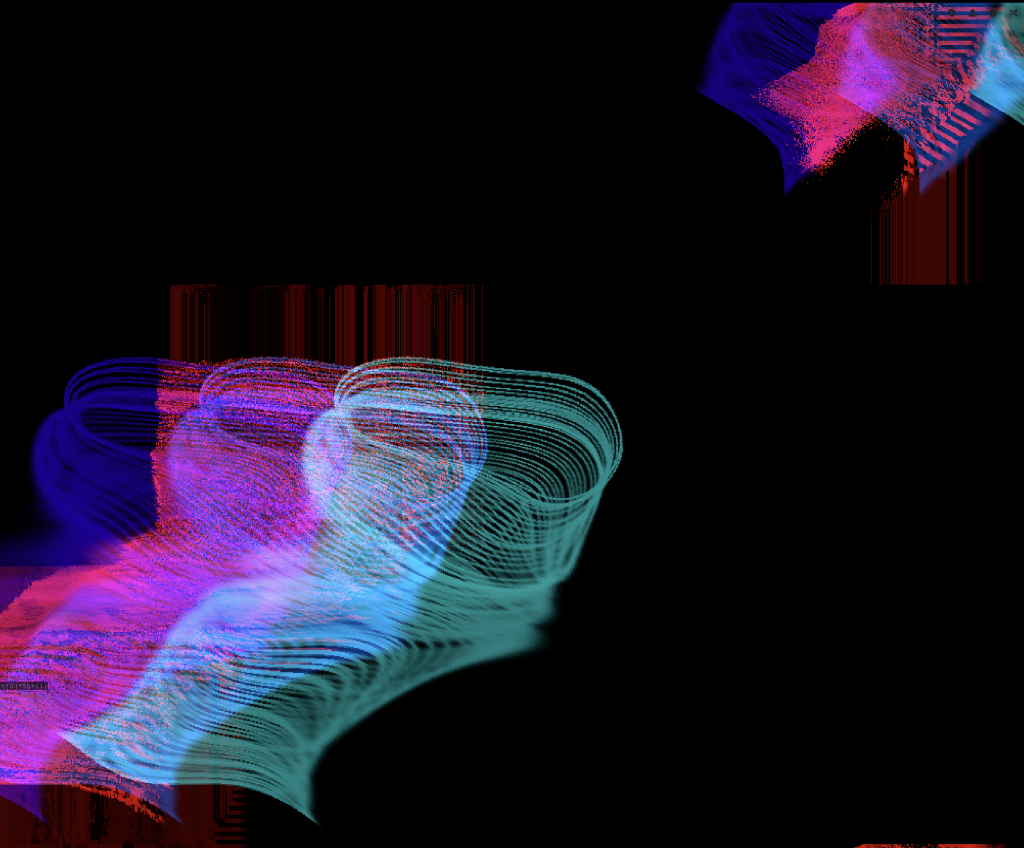
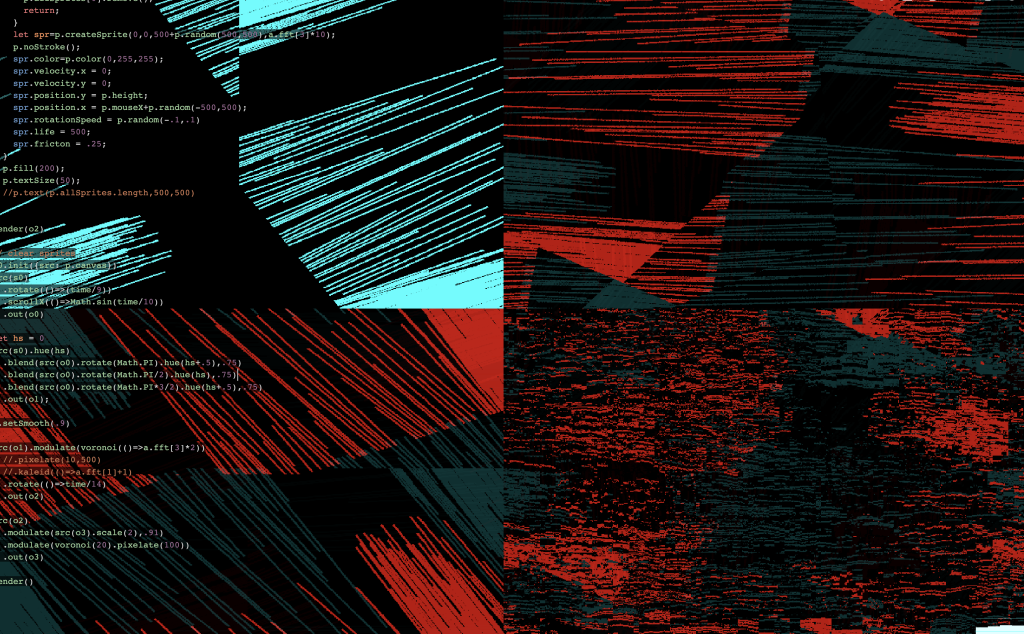
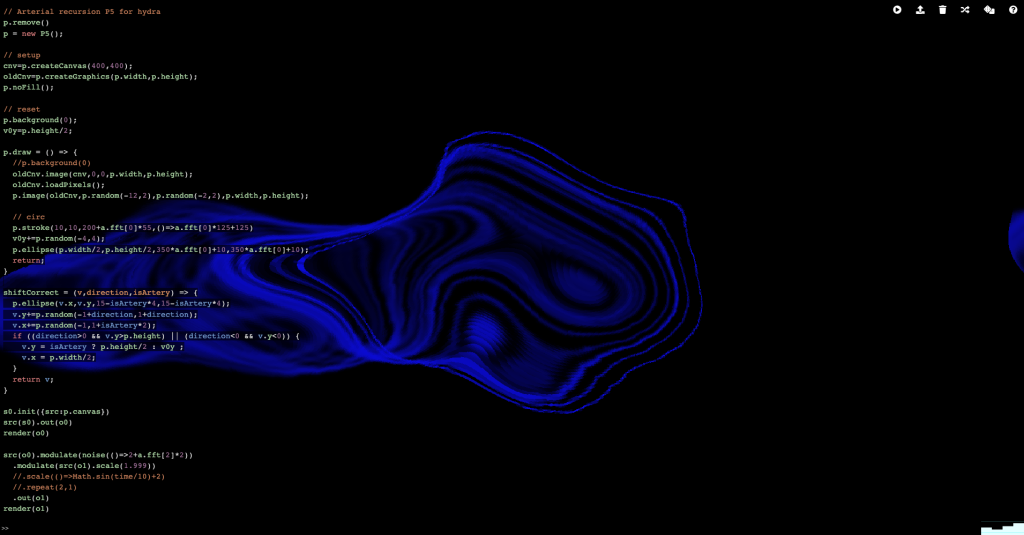
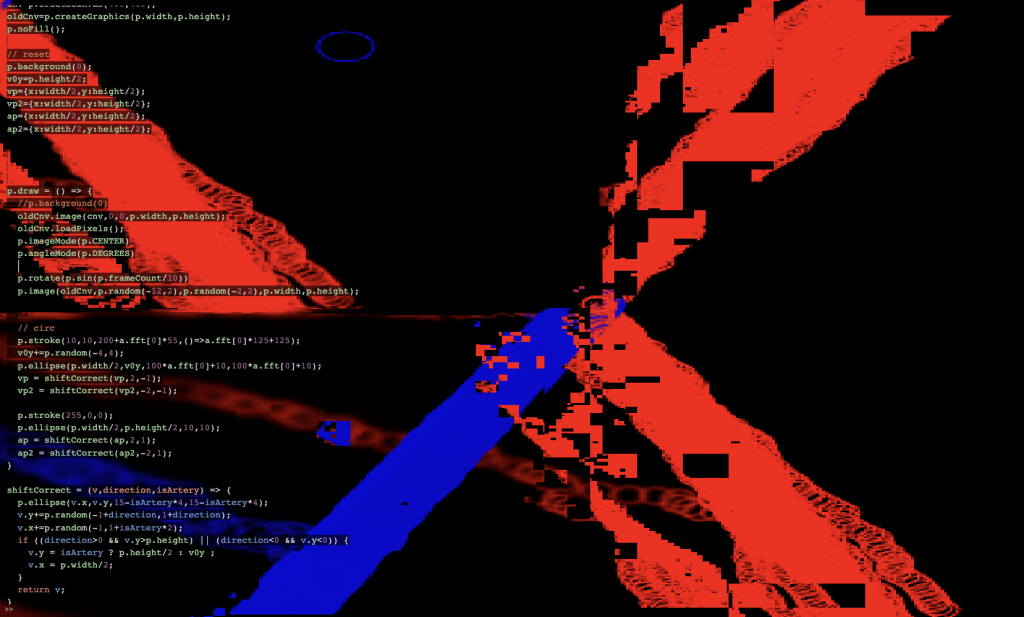
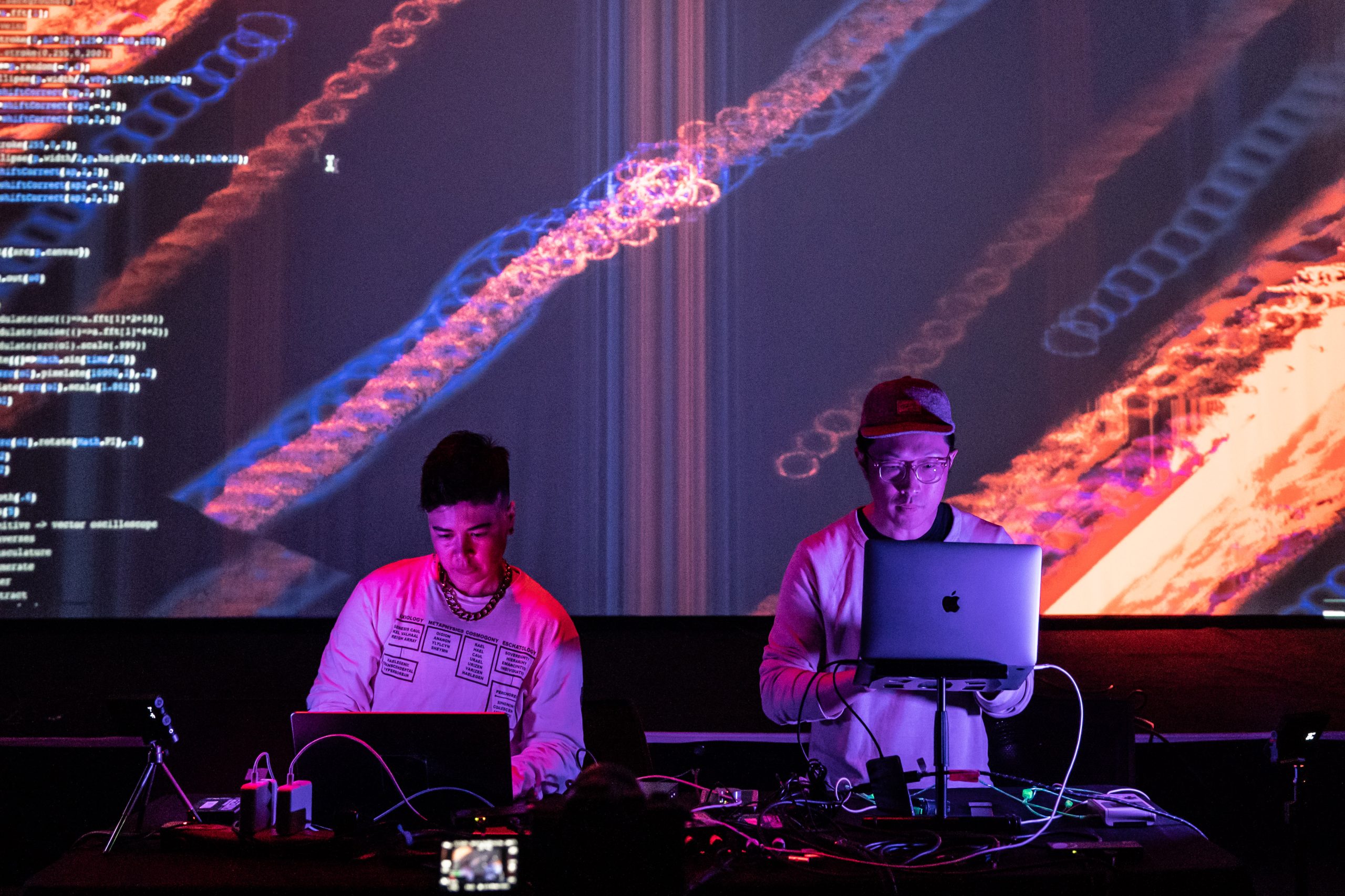
Basic canvas repetition with fft-responsivity
First time using P5 in hydra. Importing packages was slightly challenging at first, mainly because I didn’t understand the js prototype importing order at first (:x). Got p5play imported and started using box2d with some particle behavior/generation mediated by the audio.
Ended up not using this for the show since I thought it’d be challenging to switch between the canvas recursion functions I wrote and this library. P5 is extremely sensitive to errors so I wanted to limit the odds of me making a typo.
Final set starting point:
// vascular fork gen: p5 x hydra @errorbesque
p = new P5();
// setup
cnv=p.createCanvas(400,400);
oldCnv=p.createGraphics(p.width,p.height);
p.noFill();
// reset
v0y=p.height/2;
shiftCorrect = (v,direction,isArtery) => {
p.ellipse(v.x,v.y,15-isArtery*4,15-isArtery*4);
v.y+=p.random(-1+direction,1+direction);
v.x+=p.random(-1,1+isArtery*2);
if ((direction>0 && v.y>p.height) || (direction<0 && v.y<0)) {
v.y = isArtery ? p.height/2 : v0y; v.x = p.width/2;
} return v;
}
p.draw = () => {
// if ((a.fft[0]<.3 && p.frameCount%8!=0)) return;
oldCnv.image(cnv,0,0,p.width,p.height);
oldCnv.loadPixels();
p.imageMode(p.CENTER)
p.angleMode(p.DEGREES)
p.push()
p.translate(p.width/2, p.height/2);
// p.rotate(p.sin(p.frameCount/10)/4)
p.image(oldCnv,-2,p.random(-2,2),400,400);
p.pop()
p.background(0)
a0=a.fft[0];
// veins
p.stroke(0,a0*255,125+125*a0,200);
v0y=p.height/2;
p.ellipse(p.width/2,v0y,150*a0,150*a0);
}
s0.init({src:p.canvas})
src(s0).out(o0)
src(o0)
.modulate(osc(()=>a.fft[0]*2).modulate(noise(()=>a.fft[1]*2)
.out(o1)
render(o1)
// i primitive -> vector oscilloscope
// ii traverses
// iii vasculature
// iv framerate
// v faster
// vi abstract
// vii degrade+deconstruct
//1. Add noise to make vector oscillator: modulate with osc, then mod rotate with noise
//2. Start trails (uncomment background)
//3. Framerate lock (uncomment)
//4. Add objects for veins (remember to declare them all first!)
//5. Distortion -> bleed